How to use OnTriggerEnter to paste a material onto another object?
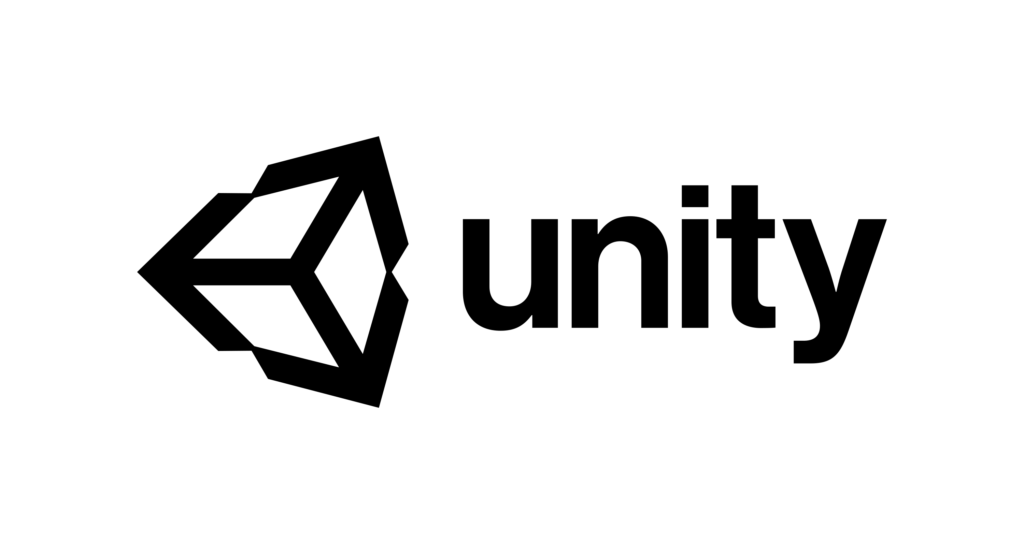
Technologies used in TETRY 3D
I’d like to start explaining the scripts from this article. I’m sure everyone is familiar with some of them, but they also serve as a sort of personal note.
Content
Preparation
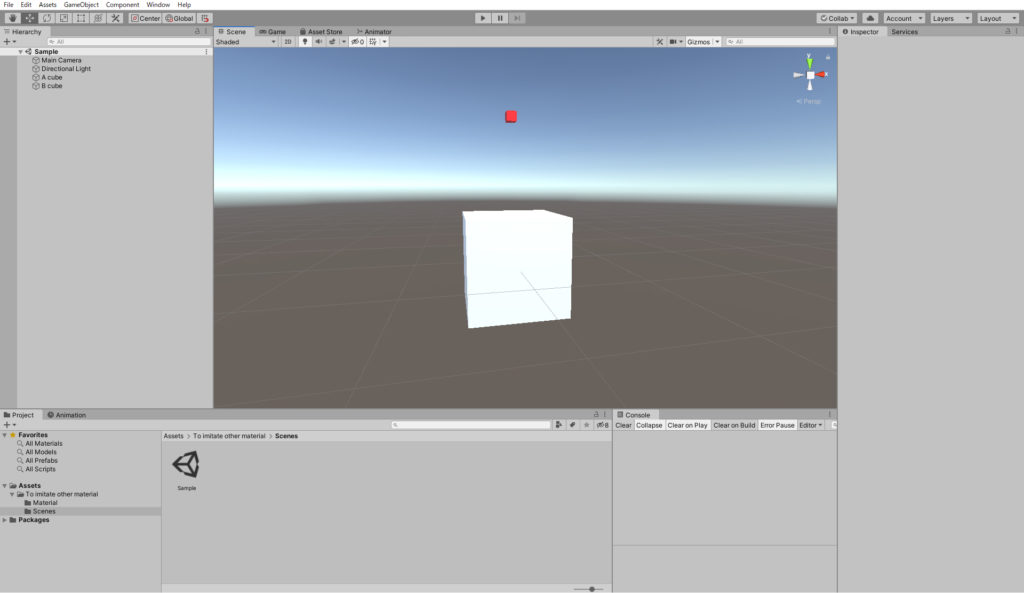
The bottom one is the recipient of the material (white) and is called “Acube”.
The top one is the moving side (red) and is named “Bcube”.
The first step is to prepare to use OnTriggerEnter.
Check the is Trigger box in the Box Collider component to receive the event.

Next, add RigidBody to the inspector of the mover.
Then I’ll create and add tags as well.
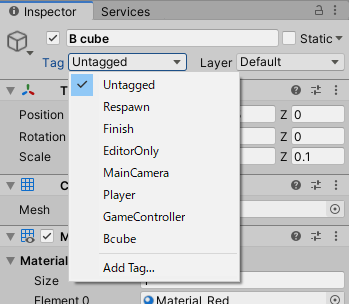
Select Add Tag and click on

Press the + button to the right of list is Enpty.

You’ll be prompted to enter a name, so I typed “Bcube” here.
Then we’ll also have a script to run Bcube.
public class move : MonoBehaviour
{
// Update is called once per frame
void Update()
{
PressX();
}
void PressX()
{
if (Input.GetKeyDown(KeyCode.X)){
Debug.Log("keyDownX");
transform.position = new Vector3(transform.position.x, 0.05f, transform.position.z);
}
}
}
Add this script to Bcube.
Implementation
The next step is to duplicate the main material.
Create the following code and paste it into Acube.
public class imitate : MonoBehaviour
{
private Renderer targetRend;
private Renderer myRend;
// Start is called before the first frame update
void Start()
{
myRend = GetComponent<Renderer>();
}
void OnTriggerEnter(Collider collider)
{
if (collider.gameObject.tag == "Bcube")
{
Debug.Log("catchB");
myRend.enabled = true;
targetRend = collider.GetComponent<Renderer>();
myRend.material = targetRend.material;
}
}
}
targetRend is for Bcube’s Renderer and myRend is for Acube.
Start() to get its own Renderer.
OnTriggerEnter is initiated when the collider of an object with a RigidBody comes into contact with it.
The argument contains the collider of the opponent.
This time, we will process only the object with the tag Bcube that we registered earlier.
In line 17, the Renderer is enabled. Even if you unchecked the Mesh Renderer in Acube beforehand, it will be visible when Bcube touches it.
In line 18, the Renderer with the information from the collider to the Bcube’s Material and so on is stored in targetRend.
In line 19, you paste the targetRend material to the myRend material.
Let’s going to try it out
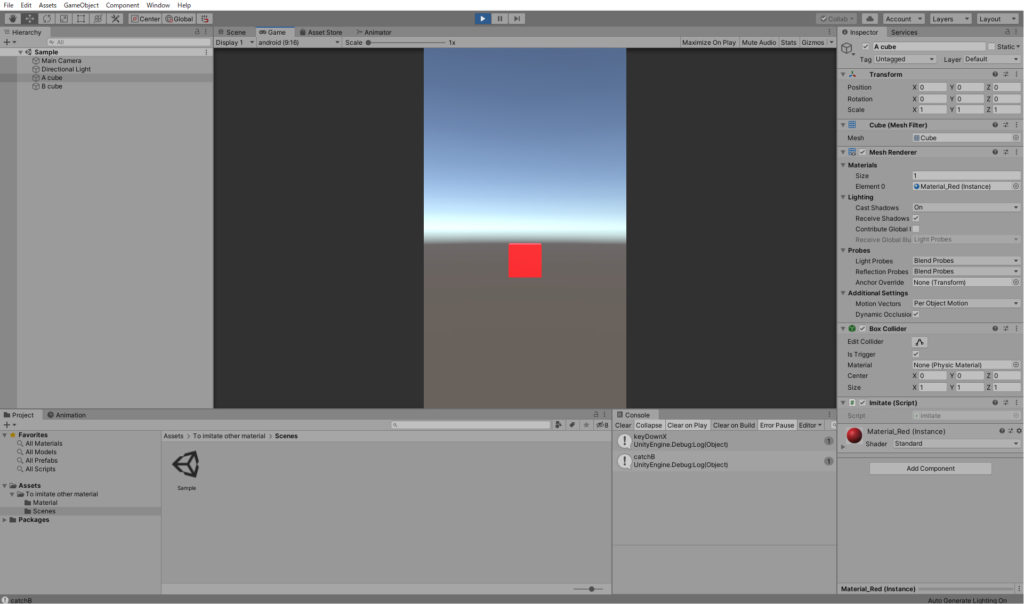
When you play the game and press the X key, Bcube will move into the Acube and apply the red material.
How to use it
This is the script I actually use in TTETRY 3D, but I use it as a basic display method. As you can see in the sample, the small blocks are the main body, and passing through the large blocks lined up makes it look like the large blocks are moving.
Apart from this, OnTriggerExit sets the reenerator back to false.
You can branch into various processes in OnTriggerStay by writing a script.
That was the technology used in the first TETRY 3D.
Thank you for your close reading.
![]() |
![]() |
- Tags:
- C#
- Commentary
- Game
0 Comments